Building Interactive Data Visualizations with D3.js
Building Interactive Data Visualizations with D3.js
D3.js is a powerful JavaScript library for creating dynamic, interactive data visualizations in the browser. When combined with React, it becomes an even more powerful tool for building modern data-driven applications.
Getting Started with D3.js in React
Before we dive into creating visualizations, let's set up our development environment and understand the basics of integrating D3.js with React components.
Installation
Start by installing D3.js: npm install d3 @types/d3
Creating a Basic Bar Chart
Let's create a simple bar chart component using D3.js and React. This example demonstrates the fundamental concepts of working with D3.js in a React environment:
1import React, { useEffect, useRef } from 'react';2import * as d3 from 'd3';34interface DataPoint {5 label: string;6 value: number;7}89interface BarChartProps {10 data: DataPoint[];11 width?: number;12 height?: number;13 margin?: { top: number; right: number; bottom: number; left: number };14}1516export const BarChart: React.FC<BarChartProps> = ({17 data,18 width = 600,19 height = 400,20 margin = { top: 20, right: 20, bottom: 30, left: 40 }21}) => {22 const svgRef = useRef<SVGSVGElement>(null);2324 useEffect(() => {25 if (!svgRef.current) return;2627 const svg = d3.select(svgRef.current);2829 // Clear previous content30 svg.selectAll('*').remove();3132 // Calculate dimensions33 const innerWidth = width - margin.left - margin.right;34 const innerHeight = height - margin.top - margin.bottom;3536 // Create scales37 const x = d3.scaleBand()38 .domain(data.map(d => d.label))39 .range([0, innerWidth])40 .padding(0.1);4142 const y = d3.scaleLinear()43 .domain([0, d3.max(data, d => d.value) || 0])44 .range([innerHeight, 0]);4546 // Create container group47 const g = svg.append('g')48 .attr('transform', `translate(${margin.left},${margin.top})`);4950 // Add bars51 g.selectAll('rect')52 .data(data)53 .enter()54 .append('rect')55 .attr('x', d => x(d.label) || 0)56 .attr('y', d => y(d.value))57 .attr('width', x.bandwidth())58 .attr('height', d => innerHeight - y(d.value))59 .attr('fill', 'steelblue');6061 // Add axes62 g.append('g')63 .attr('transform', `translate(0,${innerHeight})`)64 .call(d3.axisBottom(x));6566 g.append('g')67 .call(d3.axisLeft(y));68 }, [data, width, height, margin]);6970 return (71 <svg72 ref={svgRef}73 width={width}74 height={height}75 />76 );77};
React Integration
Remember to handle cleanup in your useEffect hook when working with D3.js to prevent memory leaks and ensure proper component lifecycle management.
Using the Bar Chart Component
Here's how to implement the bar chart component in your React application:
1import { BarChart } from './BarChart';23const App = () => {4 const data = [5 { label: 'A', value: 10 },6 { label: 'B', value: 20 },7 { label: 'C', value: 15 },8 { label: 'D', value: 25 }9 ];1011 return (12 <div className="container mx-auto p-4">13 <h1 className="text-2xl font-bold mb-4">Sales Data</h1>14 <BarChart data={data} />15 </div>16 );17};
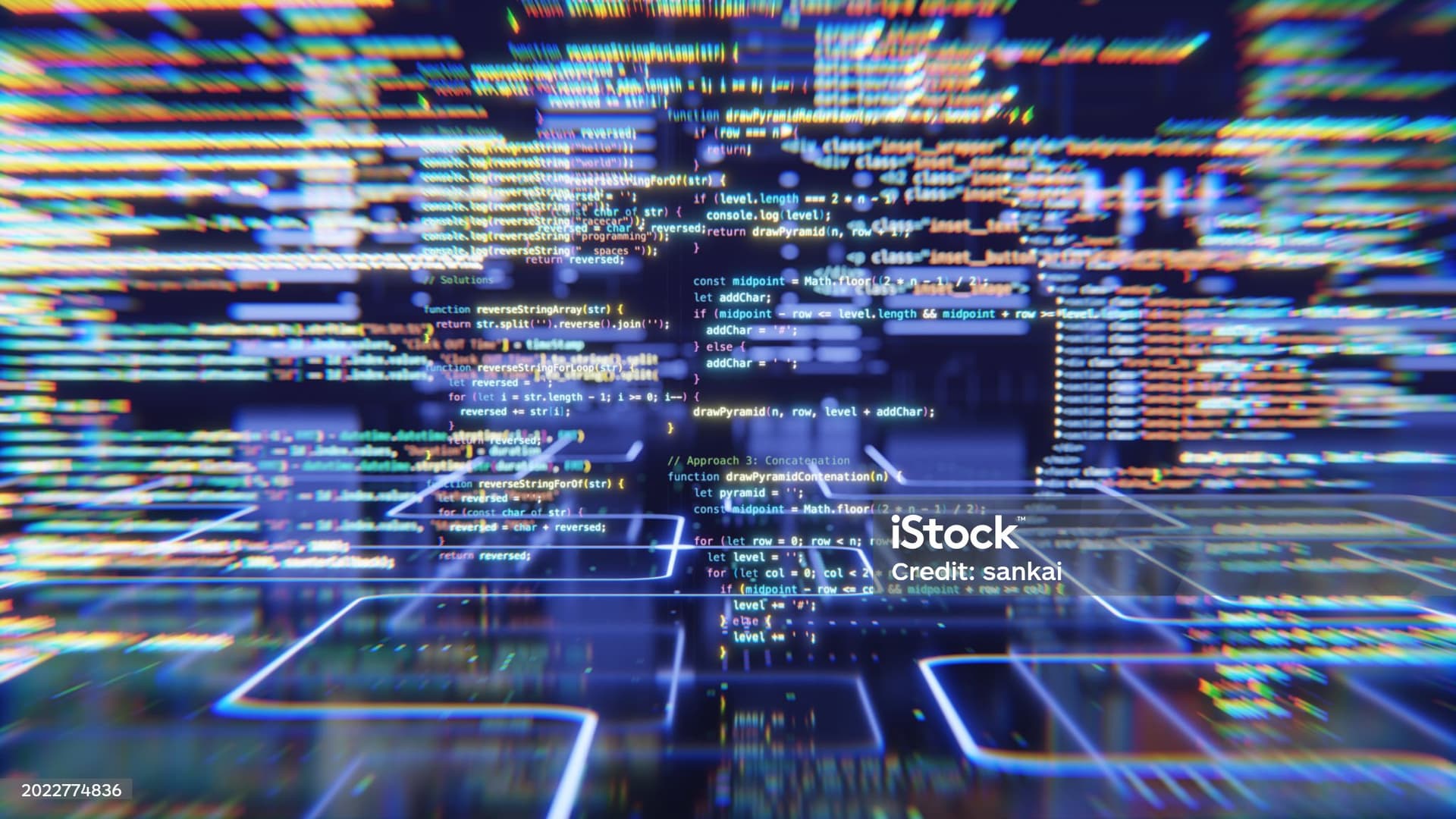
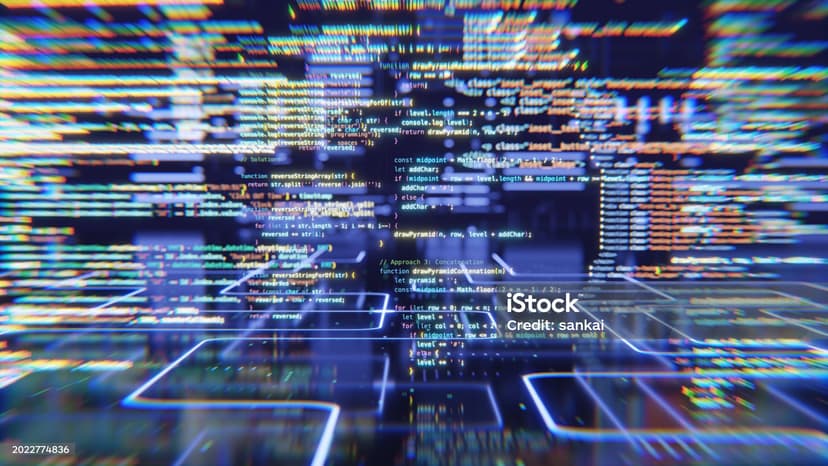