Understanding TypeScript Generics
January 15, 2025
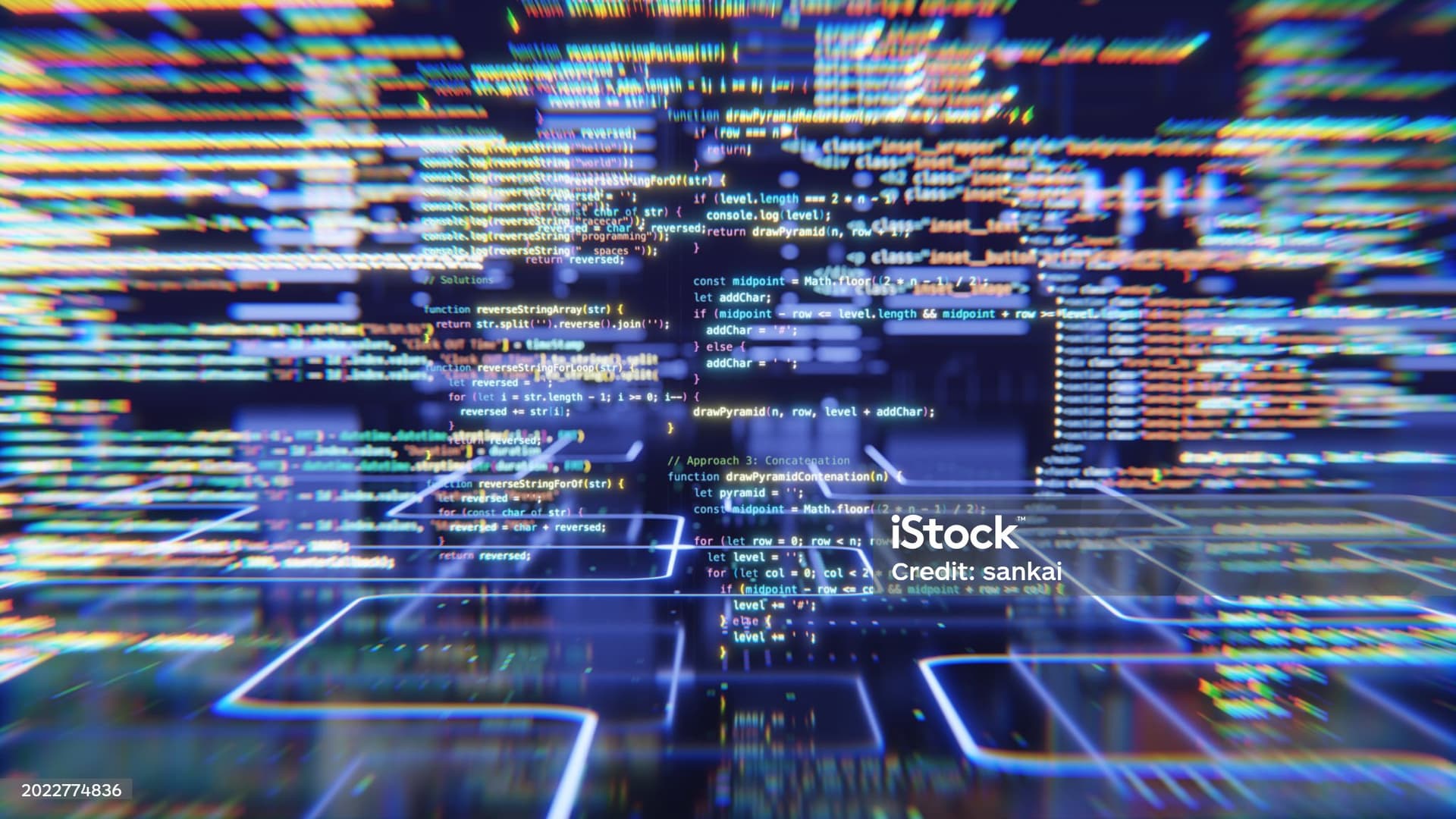
TypeScript Generics Explained
Generics are one of TypeScript's most powerful features. They allow you to write flexible, reusable code while maintaining type safety. In this comprehensive guide, we'll explore how generics work and their practical applications in real-world scenarios.
basic-generic.ts
1function identity<T>(arg: T): T {2 return arg;3}45const stringResult = identity<string>('Hello');6const numberResult = identity<number>(42);7const objectResult = identity<{id: number}>({ id: 1 });
Type Inference
TypeScript can often infer the generic type automatically. You can call identity('Hello') without explicitly specifying <string>.
Generic Interfaces
Generics are particularly useful when working with interfaces. Here's how you can create flexible, type-safe interfaces:
generic-interface.ts
1interface Repository<T> {2 get(id: string): Promise<T>;3 save(item: T): Promise<void>;4 update(id: string, item: Partial<T>): Promise<void>;5 delete(id: string): Promise<void>;6}78interface User {9 id: string;10 name: string;11 email: string;12}1314// Type-safe user repository15class UserRepository implements Repository<User> {16 async get(id: string): Promise<User> {17 // Implementation here18 }19 // Other methods...20}
Generic Constraints
Sometimes you want to limit the types that can be used with your generic function. This is where constraints come in:
constraints.ts
1interface HasLength {2 length: number;3}45function logLength<T extends HasLength>(arg: T): number {6 console.log(arg.length);7 return arg.length;8}910// These work11logLength('Hello'); // string has .length12logLength([1, 2, 3]); // arrays have .length1314// This would error15// logLength(123); // numbers don't have .length
Best Practice
Use constraints when you need to access specific properties or methods of the generic type parameter.
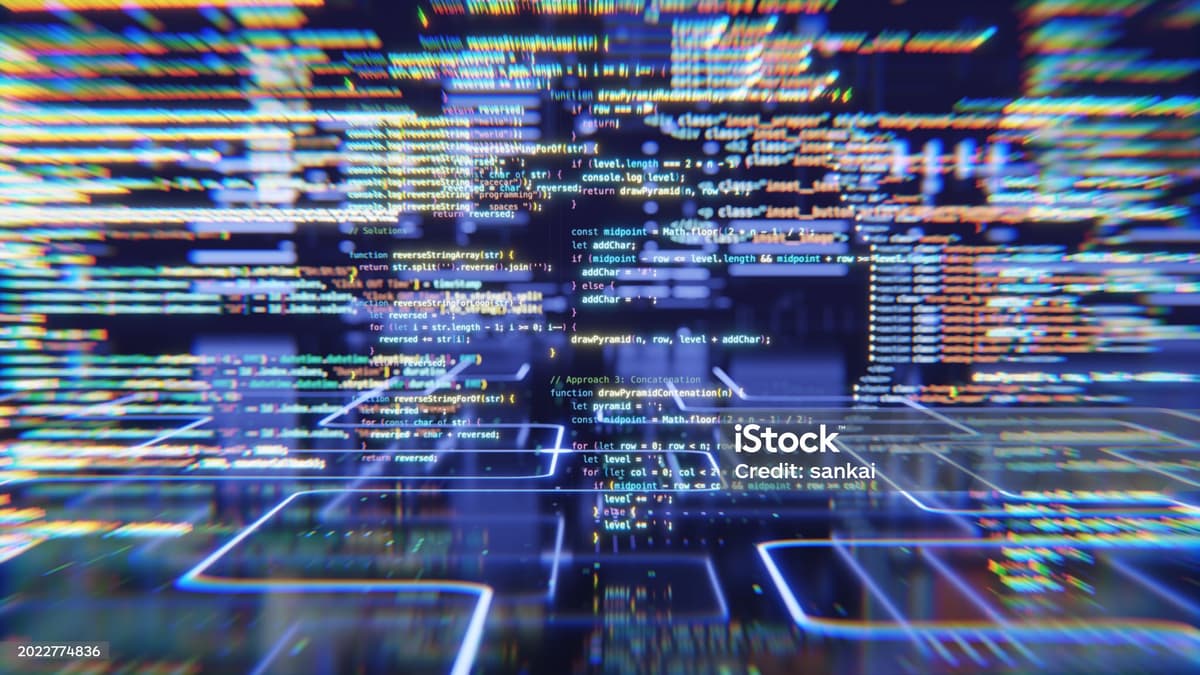